Configure client apps for social sign on
Select your platform to discover how to configure your client application to perform social sign on with an external IdP.
Configure an Android app for social sign on
Perform the following steps to configure an Android app for social sign on.
Step 1. Add the dependencies
You must add the davinci
and external-idp
modules to your project:
-
In the Project tree view of your Android Studio project, open the
build.gradle.kts
file. -
In the
dependencies
section, add the following:implementation("com.pingidentity.sdks:davinci:1.1.0") implementation("com.pingidentity.sdks:external-idp:1.1.0")
Step 2. Handle the redirect scheme
You must configure your Android app to open when the server redirects the user to the custom URI scheme you entered when setting up PingOne.
For this tutorial, the custom URI scheme is myapp://example.com
.
To configure your app to open when using the custom URI scheme:
-
In the Project tree view of your Android Studio project, open the
build.gradle.kts
file. -
In the
android.defaultConfig
section, add a manifest placeholder for theappRedirectUriScheme
property that specifies the protocol of the custom schema:android { defaultConfig { manifestPlaceholders["appRedirectUriScheme"] = "myapp" } }
Step 3. Handling IdpCollector nodes
Your app must handle the IdpCollector
node type that DaVinci sends when a user attempts to authenticate using an external IdP.
When encountering an IdpCollector
node type, call idpCollector.authorize()
to launch a custom tab and begin authentication with the external IdP:
var node = daVinci.start()
if (node is ContinueNode) {
node.collectors.forEach {
when (it) {
is IdpCollector -> {
when (val result = idpCollector.authorize()) {
is Success -> {
// When success, move to next Node
node.next()
}
is Failure -> {
// Handle the failure
}
}
}
}
}
}
Use the following parameters to the
|
The idpCollector.authorize()
method returns a Success
result when authentication with the external IdP completes successfully. If not, it returns Failure
and Throwable
which shows the root cause of the issue.
val result = idpCollector.authorize()
result.onSuccess {
// Move to next Node
}
result.onFailure {
it // The Throwable
}
The result resembles the following:
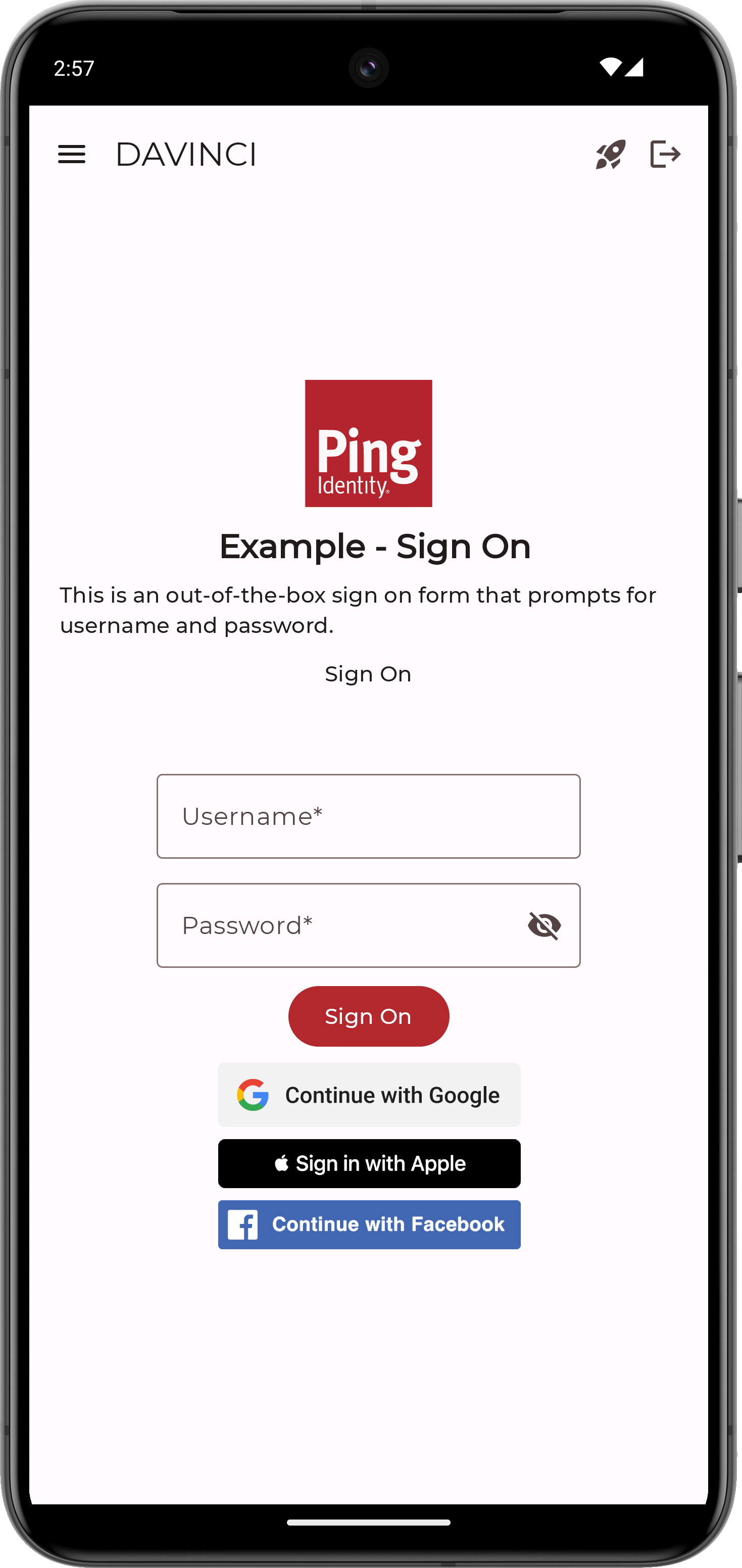
Configure an iOS app for social sign on
Perform the following steps to configure an iOS app for social sign on.
Step 1. Add the dependencies
You must add the davinci
and external-idp
modules to your project. You can use either Cocoapods or Swift Package Manager (SPM) to add the dependencies.
You can use CocoaPods or the Swift Package Manager to add the PingOne Protect dependencies to your iOS project.
Add dependencies using CocoaPods
-
If you do not already have CocoaPods, install the latest version.
-
If you do not already have a Podfile, in a terminal window, run the following command to create a new Podfile:
pod init
-
Add the following lines to your Podfile:
pod 'PingDavinci' pod 'External-idp'
-
Run the following command to install pods:
pod install
Add dependencies using Swift Package Manager
-
With your project open in Xcode, select File > Add Package Dependencies.
-
In the search bar, enter the Ping SDK for iOS repository URL:
https://github.com/ForgeRock/ping-ios-sdk
. -
Select the
ping-ios-sdk
package, and then click Add Package. -
In the Choose Package Products dialog, ensure that the
PingDavinci
andPingExternalIdp
libraries are added to your target project. -
Click Add Package.
-
In your project, import the library:
import PingDavinci import PingExternalIdp
Step 2. Handle the redirect scheme
You must configure your iOS app to open when the server redirects the user to the custom URI scheme you entered when setting up PingOne.
For this tutorial, the custom URI scheme is myapp://example.com
.
To configure your app to open when using the custom URI scheme:
-
In Xcode, in the Project Navigator, double-click your application to open the Project pane.
-
On the Info tab, in the URL Types panel, configure your custom URL scheme:
Step 3. Handling IdpCollector nodes
Your app must handle the IdpCollector
node type that DaVinci sends when a user attempts to authenticate using an external IdP.
When encountering an IdpCollector
node type, call idpCollector.authorize()
to launch an in-app browser and begin authentication with the external IdP:
public class SocialButtonViewModel: ObservableObject {
@Published public var isComplete: Bool = false
public let idpCollector: IdpCollector
public init(idpCollector: IdpCollector) {
self.idpCollector = idpCollector
}
public func startSocialAuthentication() async → Result<Bool, IdpExceptions> {
return await idpCollector.authorize()
}
public func socialButtonText() → some View {
let bgColor: Color
switch idpCollector.idpType {
case "APPLE":
bgColor = Color.appleButtonBackground
case "GOOGLE":
bgColor = Color.googleButtonBackground
case "FACEBOOK":
bgColor = Color.facebookButtonBackground
default:
bgColor = Color.themeButtonBackground
}
let text = Text(idpCollector.label)
.font(.headline)
.foregroundColor(.white)
.padding()
.frame(width: 300, height: 50)
.background(bgColor)
.cornerRadius(15.0)
return text
}
}
The idpCollector.authorize()
method returns a Success
result when authentication with the external IdP completes successfully. If not, it returns Failure
and IdpExceptions
, which shows the root cause of the issue.
Task {
let result = await socialButtonViewModel.startSocialAuthentication()
switch result {
case .success(_):
onNext(true)
case .failure(let error): //<- Exception
onStart()
}
}
The result resembles the following:
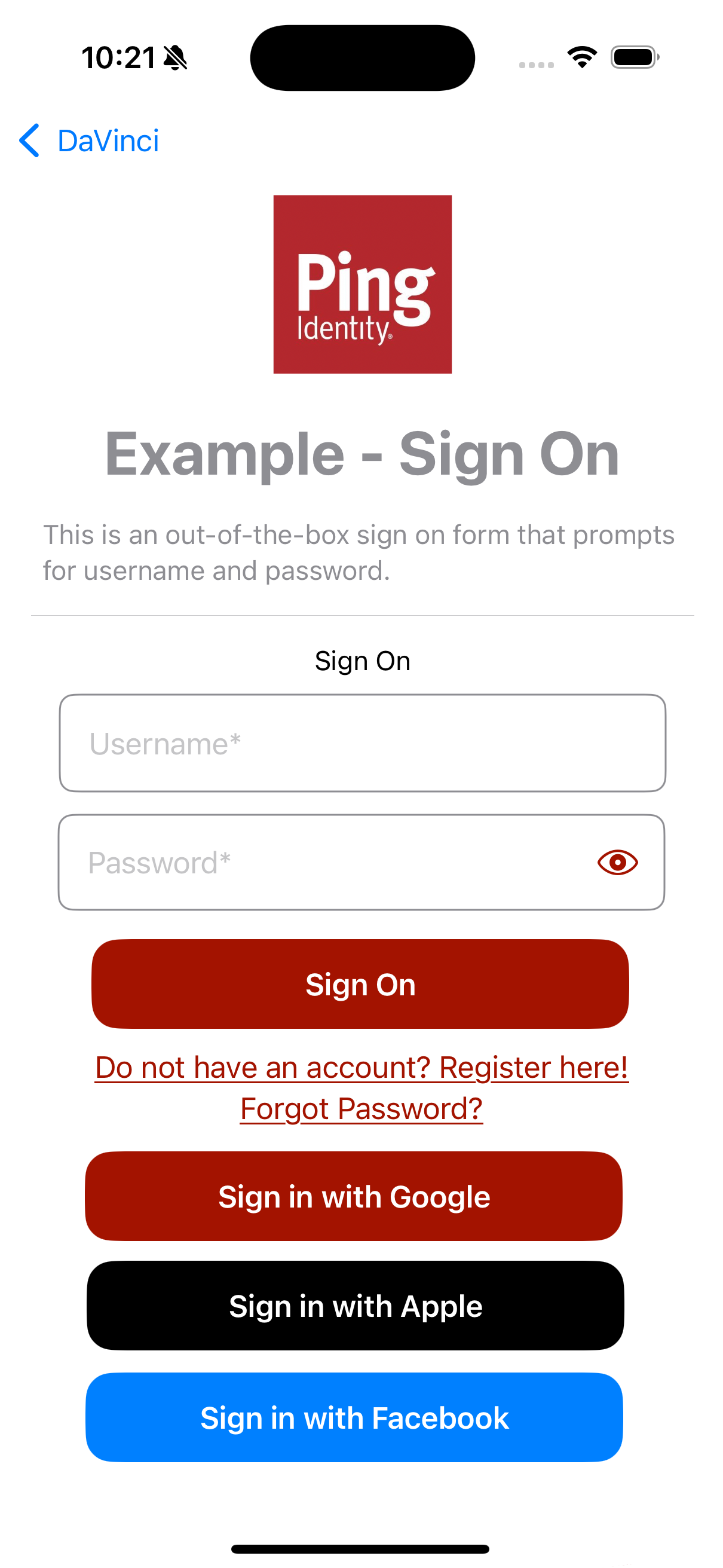
Configure a JavaScript app for social sign on
Perform the following steps to configure a JavaScript app for social sign on.
Step 1. Add the module
You must add the davinci
module to your project:
import { davinci } from '@forgerock/davinci-client';
Step 2. Handle the redirect back from the IdP
You must configure your JavaScript app to continue a flow when the server redirects the user back from the IdP.
Use the davinciClient.resume
method to continue an existing flow, rather than start a new one.
const davinciClient = await davinci({ config });
const continueToken = urlParams.get('continueToken');
let resumed: any;
if (continueToken) {
// Continue an existing flow
resumed = await davinciClient.resume({ continueToken });
} else {
// Setup configuration for a new flow
await Config.setAsync(config);
}
Step 3. Handling IdpCollector nodes
Your app must handle the IdpCollector
node type that DaVinci sends. The node contains details of the button to render and the URL, for example.
Use the davinciClient.externalIdp()
method to obtain the details from the collector:
const collectors = davinciClient.getCollectors();
collectors.forEach((collector) => {
if (collector.type === 'IdpCollector') {
socialLoginButtonComponent(formEl, collector, davinciClient.externalIdp(collector));
}
}
In this example, a socialLoginButtonComponent
handles rendering the button:
social-login-button.ts
file to render social sign-on buttonsimport type { IdpCollector } from "@forgerock/davinci-client/types";
export default function submitButtonComponent(
formEl: HTMLFormElement,
collector: IdpCollector,
updater: () => void
) {
const button = document.createElement("button");
button.value = collector.output.label;
button.innerHTML = collector.output.label;
if (collector.output.label.toLowerCase().includes('google')) {
button.style.background = 'white'
button.style.borderColor = 'grey'
} else if (collector.output.label.toLowerCase().includes('facebook')) {
button.style.color = 'white'
button.style.background = 'royalblue'
button.style.borderColor = 'royalblue'
} else if (collector.output.label.toLowerCase().includes('apple')) {
button.style.color = 'white'
button.style.background = 'black'
button.style.borderColor = 'black'
}
button.onclick = () => updater();
formEl?.appendChild(button);
}
The result resembles the following:
